Mastering Telegram Programming Code for Business Applications
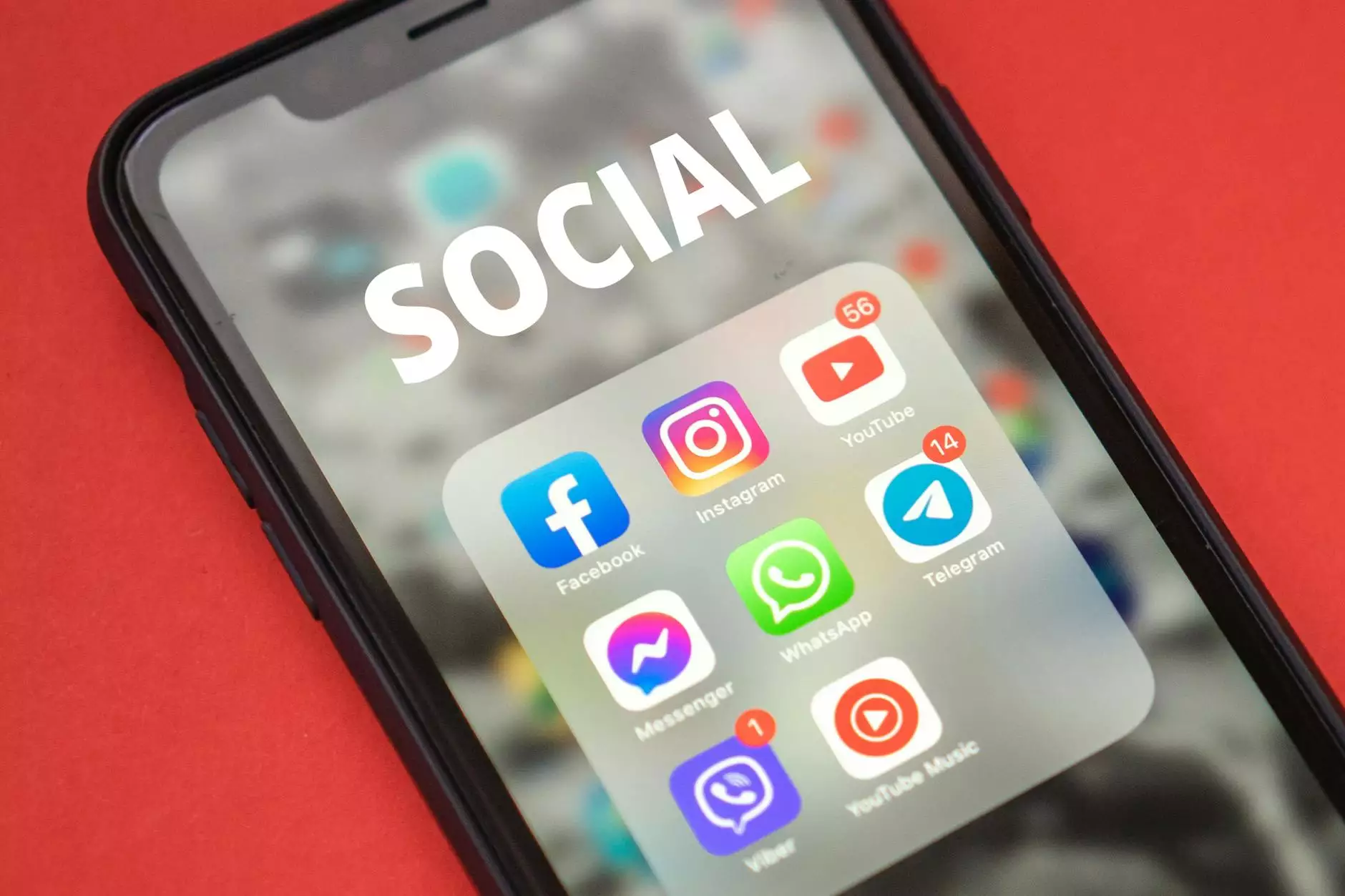
In today's dynamic technological landscape, businesses are continuously seeking innovative ways to communicate and engage with their audience. One of the most effective methods to achieve this is through platforms like Telegram. The powerful messaging app allows developers to create bots that can facilitate customer interaction, automate tasks, and improve overall business efficiency. In this article, we will delve into the world of Telegram programming code, exploring various programming languages and providing insights on how to harness this technology for your business.
Understanding the Telegram API
The Telegram API is a collection of methods and services that enable developers to interact with Telegram's functionalities. By leveraging this API, businesses can create bots that perform a wide range of tasks, such as automated responses, user engagement, and even data collection. The API supports various programming languages, with Python, JavaScript, and PHP being among the most popular choices.
The Importance of Telegram Bots in Business
Telegram bots are sophisticated tools that can significantly enhance business operations. Here are several ways they can be utilized:
- Customer Support: Automate responses to frequently asked questions.
- Marketing Automation: Send promotional messages and updates directly to users.
- User Engagement: Poll users for feedback or gather data on preferences.
- Task Automation: Schedule appointments or reminders for users.
Getting Started with Telegram Programming Code
To kick off your journey into Telegram programming code, a basic understanding of the Telegram Bot API is essential. Below are the key steps to create a simple Telegram bot using Python, one of the most favored programming languages for this purpose.
Step 1: Setting Up the Environment
Before diving into coding, ensure that you have Python installed on your system. Additionally, you will need to install the python-telegram-bot library. You can do this using pip:
pip install python-telegram-botStep 2: Creating Your Telegram Bot
To create your bot, send a message to the BotFather on Telegram. Follow these steps:
- Search for BotFather in Telegram.
- Use the command /newbot to create a new bot.
- Follow the prompts to name your bot and receive your unique bot token.
Step 3: Writing the Code
Now that you have your bot token, it's time to write the code. Below is an example implementation in Python:
import logging from telegram import Update from telegram.ext import Updater, CommandHandler, CallbackContext # Enable logging logging.basicConfig(format='%(asctime)s - %(name)s - %(levelname)s - %(message)s', level=logging.INFO) logger = logging.getLogger(__name__) # Define a command handler def start(update: Update, context: CallbackContext) -> None: update.message.reply_text('Hello! I am your bot.') def main() -> None: # Create the Updater and pass it your bot's token updater = Updater("YOUR_TOKEN_HERE") # Get the dispatcher to register handlers dp = updater.dispatcher # Register handlers dp.add_handler(CommandHandler("start", start)) # Start the Bot updater.start_polling() # Run the bot until you send a signal to stop updater.idle() if __name__ == '__main__': main()Replace "YOUR_TOKEN_HERE" with your actual Telegram bot token obtained from BotFather.
Expanding Functionality with Telegram Bots
Once you have a basic bot running, you may want to expand its functionality. Here are some advanced features to consider:
1. Inline Queries
Allow users to interact with your bot using inline queries, enabling them to receive information without directly messaging them. This can enhance user experience significantly.
2. Custom Command Handlers
Create specific commands for different functionalities. For example, users could type /help to get assistance or /info to receive details about your business.
3. Webhook Integration
For more advanced setups, consider implementing webhooks. This allows your bot to receive updates in real-time, making your application more responsive and efficient.
4. Database Integration
Integrate a database to store user information or track interactions over time, enabling you to analyze your bot's efficiency and user engagement.
Best Practices for Telegram Programming Code
To ensure your bot delivers optimal performance and user satisfaction, here are some best practices to follow:
- Maintain Clear Documentation: Document your code and provide clear instructions for users to understand how to interact with your bot.
- Regular Updates: Keep your bot updated with new features and improvements based on user feedback.
- Handle Errors Gracefully: Implement error handling to ensure the bot maintains functionality even if something goes wrong.
- Focus on User Experience: Design commands and responses with the user in mind to enhance interaction.
Conclusion
The potential of Telegram programming code extends far beyond simple messaging. By leveraging the Telegram Bot API, businesses can automate processes, enhance customer engagement, and streamline operations effectively. As the demand for sophisticated communication tools increases, understanding and utilizing Telegram's features will position your business at the forefront of innovation. Start today, and unlock new avenues for success with your very own Telegram bot!
Further Resources
For those eager to learn more about developing Telegram bots or improving their existing ones, consider exploring the following:
- Official Telegram Bot API Documentation
- Python Telegram Bot GitHub Repository
- Tutorials Point - Telegram Bots
- FreeCodeCamp - Create a Telegram Bot with Python